So you've heard about it before, but you're wondering, "What does refactoring code mean?". Refactoring code is the process of restructuring existing computer code without altering its external behavior. It's an important part of software development because it helps keep a codebase organized, maintainable, and less error-prone over time. Refactoring code can also make it easier to add new features or fix bugs in the future. There are benefits and drawbacks to refactoring code, but despite any of the risks, refactoring is an essential part of keeping your codebase healthy.
In this guide for aspiring software engineers, I'll share my thoughts on the proper way to refactor code, offer best practices for ensuring success, and explore when to start refactoring your code. By the end of this guide, I hope that you'll have a better understanding of how to refactor code for long-term success.
Benefits of Refactoring Code
Refactoring code has numerous benefits that outweigh its drawbacks when done properly. It can lead to improved readability, maintainability, and extensibility of the codebase. Refactored code is typically much easier to understand and follow than its original version -- which makes sense if you consider we're going to take the time to improve it while we're in there. By breaking down large chunks of code into smaller, more manageable parts, it becomes easier to maintain and extend as time goes on.
Improved Readability
When refactoring code, we break down large and complex code blocks into smaller, more manageable blocks. This often leads to improved readability, as the code becomes easier to read and follow. Refactored code is often more concise and straightforward, which makes it easier for developers to understand what is happening at any given point in the code. If you consider how much time we spend reading code vs writing code, then you'll know that there's a great deal of value in readable code.
Maintenance and Extensibility
Refactored code is much easier to maintain and extend, as it's often better organized and more modular. This makes it easier to add new features or fix bugs in the codebase without introducing new issues. Refactored code is also more scalable than its original version, which means that it can be extended to meet new requirements as needed.
Of course, you need to be refactoring code with these goals in mind. If you refactor code to simplify renaming and readability as a priority, you will not necessarily introduce more flexible code.
Drawbacks of Refactoring Code
There are also some drawbacks of refactoring code. One of the main issues is the potential introduction of bugs, which can happen if the refactoring is not done correctly. This is especially likely if the area of code is brittle to begin with and has low test coverage. Another issue is that refactoring can be a time-consuming process, especially when the codebase is quite large. Developers must weigh the benefits and drawbacks of refactoring when deciding whether or not to refactor code.
Despite the potential drawbacks, we must remember that the long-term benefits of refactoring code often outweigh the short-term costs. By investing time and effort into properly refactoring our code, we create a more stable and maintainable codebase that is much easier to work with. As with any software engineering practice, there are pros and cons to refactoring code, but in most cases, the benefits make it a worthwhile practice. Without refactoring, we'd be faced with eventually abandoning code altogether or rewriting it from scratch.
Potential Introduction of Bugs
One of the main drawbacks of refactoring code is the potential introduction of bugs. When code is refactored, it can be easy to introduce new bugs if the process is not done correctly. This can lead to issues down the line and cause unnecessary headaches for developers and their teams.
Time Constraints
Refactoring code can be a time-consuming process, especially if the codebase is large and complex. Developers must weigh the benefits of refactoring against the time it takes to do so. While refactoring can be time-consuming, it's often worth the investment in the long run. Properly refactored code is typically much easier to maintain and extend in the future, which can save time over the long term.
Given that often refactoring can be done incrementally, I highly advise leaning into this. Set some goals upfront for your refactoring. Identify some milestones. If you additionally layer in an element of time-boxing, you can pull the metaphorical ripcord early as needed so you don't go off the rails refactoring.
How to Refactor Code
When planning to refactor code, there are several steps you should take beforehand to ensure a smooth and effective process. One essential step is writing tests to ensure the refactored code still works as intended. Another is understanding the code you are refactoring to identify any dependencies or potential pitfalls. Writing tests ahead of time can be easier said than done though if the entire point of refactoring was to make something more testable.
To illustrate the process of code refactoring, let's examine an example of unrefactored code. Imagine you're working on a program that calculates the cost of an order, and the code is littered with repetitive if-else statements. This code is hard to maintain and not very readable, making it an ideal candidate for refactoring.
There are various ways to refactor code, depending on the situation. In this case, to simplify the conditional statements, we can use methods like "extract method" and "replace conditional with polymorphism." These methods enable us to simplify the code and make it more maintainable. There are numerous other methods we can leverage in refactoring, each attempting to help us with different goals.
To ensure your refactoring goes smoothly though, I do highly recommend you think about how you'll use coded tests to verify things are as you expect. You can start by creating a test suite that captures the current behavior. Then refactor small sections of code and run tests frequently to ensure no errors arise. If you can't get tests in place ahead of time, I highly advise that you proceed very cautiously.
Best Practices for Refactoring Code
Despite the benefits of refactoring code, certain mistakes can cause harm to your codebase. One such mistake is over-engineering, which refers to overcomplicating the code and creating unnecessary additional functionality. To avoid over-engineering and over-refactoring, ensure that you keep the original functionality intact and focus on simplifying the code rather than adding new features. This is incredibly difficult for a lot of us, I get it, so just continue to drive this to the front of your mind as you work.
Another tip to keep in mind is refactoring small sections of code at a time. This approach can make the process less daunting and easier to manage. I mentioned earlier that working towards milestones is very beneficial, so consider how your refactoring can be done in increments. Additionally, frequent testing can help catch bugs early on in the process, saving time and resources in the long run.
Are you interested in boosting your refactoring skills? Check out my course on Dometrain:
Refactoring for C# Devs: From Zero to Hero
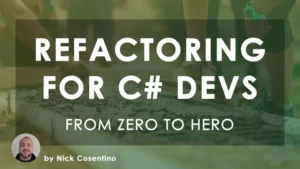
When to Refactor Code
Refactoring code is an important practice, but it can also be time-consuming. So, how do you know when code is a good candidate for refactoring? Here are some signs that indicate your code could benefit from being refactored:
- Hard-to-maintain code: If your code has become difficult to update or fix bugs in, it may be a sign that it needs to be refactored. This could be due to overly complex code, poor design patterns, or even outdated libraries or frameworks.
Hard-to-understand code: If anyone, including yourself, has trouble understanding your code, it might be a sign that it needs to be refactored. This could include confusing naming conventions, scattered or disorganized code, or even just poorly written documentation.
In addition to these signs, here are some more specific scenarios that might warrant some refactoring effort:
Adding new features: When you're adding new features to an existing codebase, you may find that the code's architecture doesn't support the new functionality you're trying to add. Refactoring code can help you create a more flexible and scalable architecture that can better handle future updates.
Fixing bugs: While it may be tempting to just patch up bugs as they're discovered, this can lead to a codebase that's difficult to maintain over time. Refactoring the code that contains the bugs can help address the root cause of the issue, rather than just fixing the symptoms. This is especially the case if the cause of the bug was ambiguity in how the code was supposed to behave.
I'd like to point out something very important though: being a good candidate for refactoring does NOT mean it's a priority to refactor it. Please weigh the priority of the refactoring effort against your other deliverables, such as features and bug fixes.
Other Considerations for Refactoring Code
It's important to remember that refactoring code is not a solo activity. Teamwork and communication play important roles in effective code refactoring. When performing code refactoring, it's important to make sure that the team is aware of what is happening. This helps prevent duplication of effort and ensures that everyone is working towards the same goal. Additionally, team members can provide valuable insights and suggestions for how to improve the refactoring process. This can lead to better code quality and more efficient workflows.
In addition to teamwork and communication, there are several books and online tutorials available for further reading and learning about code refactoring:
- "Refactoring: Improving the Design of Existing Code" by Martin Fowler is a classic book on the subject and provides practical guidance on how to refactor code.
- "Working Effectively with Legacy Code" by Michael Feathers
- "Clean Code: A Handbook of Agile Software Craftsmanship" by Robert C. Martin.
I also have my very own course on Dometrain: Refactoring for C# Devs which can help you understand tools and techniques for refactoring.
Are you interested in boosting your refactoring skills? Check out my course on Dometrain:
Refactoring for C# Devs: From Zero to Hero
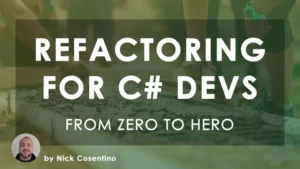
Answering What Does Refactoring Code Mean
Together, we discussed the benefits of refactoring code, such as improved readability, maintainability, and extensibility, while also delving into the drawbacks, such as potential bugs and time constraints. We also walked through practical steps on how to refactor code, including tips for avoiding common mistakes and best practices to follow.
It's important to remember that code refactoring is an essential practice to ensure that your codebase remains efficient and effective. By staying up-to-date on software engineering best practices, including code refactoring, you can continue to develop your programming skills and stay competitive in the industry.
Remember to check out my course on Dometrain: Refactoring for C# Devs if you want to skill up on your refactoring! If you're interested in more learning opportunities, subscribe to my free weekly newsletter and check out my YouTube channel!
Affiliations
These are products & services that I trust, use, and love. I get a kickback if you decide to use my links. There’s no pressure, but I only promote things that I like to use!
- BrandGhost: My social media content and scheduling tool that I use for ALL of my content!
- RackNerd: Cheap VPS hosting options that I love for low-resource usage!
- Contabo: Affordable VPS hosting options!
- ConvertKit: The platform I use for my newsletter!
- SparkLoop: Helps add value to my newsletter!
- Opus Clip: Tool for creating short-form videos!
- Newegg: For all sorts of computer components!
- Bulk Supplements: Huge selection of health supplements!
- Quora: I answer questions when folks request them!