Refactoring legacy code can be a challenging task for any software engineer. Believe me -- I've been there, even in situations where I was the author of said legacy code.
Legacy code refers to source code that is outdated, often encoded in older programming languages, or with outdated techniques that are no longer in use. This type of code can pose a challenge when it comes to implementing new features, updating applications for security and performance improvements, and maintaining readability. Legacy code can be difficult to work with due to the nature of the code being outdated or written poorly, which can extend to the overall complexity of the codebase and make it difficult to understand and update.
In this article, I'll share some best practices for effectively refactoring legacy code. By following these best practices, software engineers can increase their software's longevity, maintainability, and reliability. It's important to place emphasis on effective refactoring, as failure to do so can lead to a codebase with a high level of technical debt, negatively impacting performance and the overall success of the project. Nobody wants that.
Understanding Legacy Code
Legacy code refers to the code that has been inherited from a previous system, often written in an outdated programming language, or framework that may no longer be supported. It can be challenging to understand and maintain, making it difficult for software engineers to work with.
To effectively refactor legacy code, it's essential to understand its common characteristics, challenges, and limitations.
Common Characteristics of Legacy Code
There are a ton of characteristics of legacy code that folks will refer to, but some of the most common include a lack of documentation, unclear code structure, and the use of deprecated or outdated libraries and frameworks. Other characteristics of legacy code may include inconsistent coding conventions, low test coverage, and convoluted control and data flow.
These characteristics make legacy code difficult to decipher, maintain, and modify. Without documentation and test coverage, engineers may struggle to understand how the code works and what the intended behavior is. The unclear structure may make it challenging to figure out how different pieces relate to each other, and the use of deprecated or outdated libraries may lead to conflicts or incompatibilities with newer tools and platforms.
Despite these challenges, it's crucial to understand legacy code before attempting to refactor it. Properly understanding legacy code is essential to create a plan for refactoring that is effective and efficient. By acknowledging the challenges and limitations that come with legacy code, software engineers can develop better strategies for working with it.
Before You Start Refactoring
As software engineers, many of us are instructed that making "boy scout" changes along the way when you're in a codebase is helpful. While I agree with the idea of prioritizing and not neglecting to pay down technical debt, I disagree with the idea of refactoring in line with your other deliverables. Especially if it's in a known legacy area of the code.
It's essential to prepare before diving straight into refactoring legacy code. Preparation helps ensure that you don't accidentally introduce new bugs or issues into the codebase. You also don't want to go down a rabbit hole refactoring for extended periods of time. Before starting, you should have a thorough understanding of the codebase and identify the areas that require refactoring.
When preparing to refactor legacy code, it's extremely helpful to start with test coverage to ensure that the code still works correctly afterward. It's also important to identify refactoring opportunities and prioritize the areas of code that require the most attention. By doing this, you can work through the codebase more effectively, reducing the potential for issues down the line.
Identifying Opportunities for Refactoring
Identifying opportunities for refactoring is crucial to making the process more efficient and effective. When identifying areas of the codebase that can benefit from refactoring, it's essential to analyze the code and look for things such as duplicated code, unused variables, and methods that could be combined or separated. These are typically areas that could use fine-tuning to be more efficient.
Another area to focus on is code that is causing performance-related issues. This code is often restricting system performance and can be significantly improved by refactoring. By identifying these areas in advance, you can reduce technical debt and ensure that your code remains maintainable.
Ultimately, the key to effective refactoring is taking the time to prepare and identify the areas that need attention. This preparation helps ensure that you are able to work through the code more effectively, while also reducing the potential for introducing new bugs or issues into the codebase.
Opportunity != Priority
But this is the important part that I want you to realize. Just because there's an opportunity to refactor something does *NOT* mean that it is a priority to do so. I think this is one of my biggest arguments in the debate about refactoring and addressing tech debt.
This stance stems from two major things:
- On multiple occasions, I've seen programmers decide (on their own) that something needed to be refactored. They decide the scope of that refactoring (on their own) and start making claims that the refactoring blocks other deliverables. If they're working with a project manager, product owner, or other role that isn't as technical enough to challenge them (and other programmers aren't), then it gets blessed. Often this happens because they've already started and they're already so invested... and there's just insufficient backbone from other stakeholders to push back and say "But what's the priority of doing this? What's the business value?"
- Individuals who want to "boy scout" their changes and commit their changes alongside their other work. The other work has a regression, so now they're also rolling back their tech debt fixes. Or they they failed to realize just how bad the test coverage was in the legacy area and now their feature is getting rolled back as well.
I think it's absolutely CRITICAL that teams invest in addressing tech debt, but I strongly believe you need to work to prioritize it with your other work.
Are you interested in boosting your refactoring skills? Check out my course on Dometrain:
Refactoring for C# Devs: From Zero to Hero
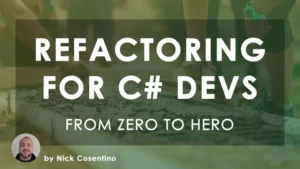
Best Practices for Refactoring Legacy Code
As a software engineer, it's inevitable that you'll come across legacy code at some point. Refactoring legacy code can be a daunting task, but using best practices can make the process run more smoothly and effectively. In this section, together we'll explore some of the best practices for refactoring legacy code.
Coding Standards for Refactoring
When tackling legacy code, it's important to have a clear set of coding standards in place. Coding standards can help you maintain consistency throughout the codebase, which is especially important when working with older code.
In practice, coding standards can include things like naming conventions, commenting and documentation requirements, and testing criteria. Having a clear set of coding standards can make code review easier and can help ensure that code is maintainable over the long term.
If you're about to go making changes, you should be up to speed on what the latest conventions and patterns are. There's no sense in refactoring this code and reintroducing patterns that are no longer used in the codebase.
Testing is Critical for Refactoring
Testing is an essential part of the refactoring process. Before you begin refactoring, it's important to make sure you have a clear understanding of how the code is currently working. Once you've refactored the code, you'll need to run all of those tests to ensure that it still works as expected.
Oh -- But there are no tests? Or there are a couple of poorly written tests or only a couple of unit tests over the code that you inevitably have to delete? This should ring some warning bells. You'll want to see if you can write some functional tests over the code or otherwise have a way to validate the behavior of what you're about to refactor.
Best practices for testing after refactoring include creating a suite of unit tests that cover critical areas of the codebase and ensuring that all tests pass before committing any changes. It's also important to test the code in a production-like environment to ensure that the changes will work in the real world. You don't want to do all of that work to find yourself defending a commit saying, "Well, it worked on my machine".
Remember to take your time, stay organized, and prioritize reducing technical debt to make sure you're making the most of your refactoring efforts.
Overcoming Common Challenges
One of the biggest challenges in refactoring legacy code is dealing with dependencies. Often, legacy code has dependencies that are outdated or no longer needed, making it difficult to refactor without breaking other parts of the system. To overcome this challenge, it's important to take a strategic approach to handling dependencies.
Approaches for Handling Dependencies
One approach is to identify and remove any unnecessary dependencies in the code. This can be done by analyzing the code and determining which dependencies are necessary for the functionality of the program. Once these dependencies have been identified, any unnecessary ones can be safely removed.
Another approach is to isolate the dependencies and create interfaces or abstractions to make them more manageable. This helps to reduce the impact of dependencies when making changes to the code. Refactoring towards these abstractions can sometimes be done gradually, by refactoring small or isolated parts of the system at a time.
However, it's important to keep in mind that minimizing dependencies is not always possible or practical. In these cases, it's important to have a solid understanding of how these dependencies relate to each other and how changes in one part of the system will affect other parts.
Other Common Challenges When Refactoring Legacy Code
Other common challenges in refactoring legacy code include:
- Identifying the right places to refactor
- Understanding the codebase
- Ensuring proper testing before and after refactoring
Of this list, I think that the first one can be greatly simplified for folks. Instead of looking for the areas to refactor, pay attention to where you're working and where you have upcoming work. If you're working in areas that feel brittle (i.e. you keep having regressions) or you find the code is hard to navigate in the first place... it might be a good opportunity to refactor.
Simply digging up some old code that hasn't been touched in 7 years to point out the flaws isn't super helpful if it's currently doing its job. If there are no reports of bugs and no scheduled work in that area, introducing any amount of risk just to clean it up would need some other type of justification.
Are you interested in boosting your refactoring skills? Check out my course on Dometrain:
Refactoring for C# Devs: From Zero to Hero
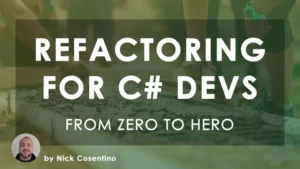
Wrapping Up Refactoring Legacy Code
Refactoring legacy code can be a daunting task for software engineers due to the complex nature of such systems. However, following best practices can ease the process and lead to better quality code. Understanding legacy code and identifying refactoring opportunities are crucial first steps. Preparing for refactoring through testing and identifying challenges can help engineers avoid common pitfalls.
By following the best practices outlined in this article, I hope that you can more effectively refactor legacy code while minimizing technical debt. Remember, refactoring and reducing technical debt is important, but so is delivering value to customers. Ensure that you are balancing the two and justifying your refactoring efforts with business value.
Remember to check out my course on Dometrain: Refactoring for C# Devs if you want to skill up on your refactoring! If you're interested in more learning opportunities, subscribe to my free weekly newsletter and check out my YouTube channel!
Affiliations
These are products & services that I trust, use, and love. I get a kickback if you decide to use my links. There’s no pressure, but I only promote things that I like to use!
- BrandGhost: My social media content and scheduling tool that I use for ALL of my content!
- RackNerd: Cheap VPS hosting options that I love for low-resource usage!
- Contabo: Affordable VPS hosting options!
- ConvertKit: The platform I use for my newsletter!
- SparkLoop: Helps add value to my newsletter!
- Opus Clip: Tool for creating short-form videos!
- Newegg: For all sorts of computer components!
- Bulk Supplements: Huge selection of health supplements!
- Quora: I answer questions when folks request them!