As software engineers, it is essential to write and maintain code that is easy to understand and maintain. Simplicity in code is critical because it reduces the mental overhead needed to digest code. Control flags are commonly used in software development, but they can add complexity to the code. Control flags are variables that control the behavior of a program. They are used to stop or continue executing a piece of code. The drawback of control flags is that they can make the code more challenging to read and follow. Luckily we can use remove control flag refactoring to help us out!
The remove control flag refactoring technique eliminates the dependence on control flags. By removing control flags, the code becomes simpler and clearer to read. This technique involves changing existing control statements to take advantage of the early exit feature. The result is usually code that has fewer nested statements and is easier to follow.
There are many benefits of applying the remove control flag refactoring technique. It can make code easier to understand and maintain, which is particularly important for large codebases. Additionally, it can reduce the number of bugs present in code by removing unnecessary complexity. By applying this technique, software engineers can produce code that is easier to work with both for themselves and their colleagues.
The Remove Control Flag Refactoring
Remove control flag refactoring is a technique used to simplify code and eliminate unnecessary control flags. The traditional approach to using control flags can be cluttered and challenging to maintain. Remove control flag refactoring replaces the control flags with clearer code constructs, leading to increased readability and understandability.
The Traditional Approach to Using Control Flags
Control flags are variables that are used to control the flow of a program. They are used to manage the execution of a program by telling it whether to move on to another section of code or continue in its current direction. They are commonly used in loops and conditional statements to determine when to exit or continue to execute a block of code. Here is an example of a traditional approach to using control flags in C# code:
bool endOfData = false;
while (!endOfData) {
// do some code here
if (condition1) {
endOfData = true;
}
else if (condition2) {
// do something else here
}
else {
// do some other code here
}
}
The Remove Control Flag Refactoring Technique
Remove control flag refactoring is a technique that replaces a control flag with return statements or break/continue statements. Instead of using the control flag to break out of the loop or condition, the Remove Control Flag technique uses these return and break/continue statements to directly exit the loop. Here is an example of remove control flag refactoring in C# code:
int index = 0;
while (index < items.Count) {
// do some code here
if (shouldStop()) {
break;
}
else {
index++;
}
}
As seen above, the modified code removes the control flag with the break
statement, making it easier to understand and read. This new approach is more explicit about the exit condition and removes the need for additional code constructs that might obscure the intent.
By simplifying the code, remove control flag refactoring improves code maintainability and readability by eliminating unnecessary control flags.
Are you interested in boosting your refactoring skills? Check out my course on Dometrain:
Refactoring for C# Devs: From Zero to Hero
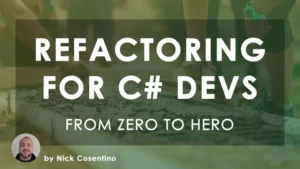
Applying the Remove Control Flag Refactoring
When applying the remove control flag refactoring, there are specific steps that need to be taken. These steps include identifying the control flag, changing the control flag, and simplifying the code. Below is a step-by-step guide to applying the Remove Control Flag Refactoring technique in programming.
Step 1: Identifying the Control Flag
The first step is identifying the control flag in the code. Control flags often appear in code as boolean variables that are used to control the flow of a program by changing their value in response to logic in the code. A control flag can also be a variable that is used within a loop to determine when the loop should stop. One should identify such variables and try to apply the remove control flag refactoring technique if an opportunity arises.
An example of identifying the control flag in C# code could be a variable such as isComplete
that is used as a condition to control the flow of a loop.
Step 2: Changing the Control Flag
The second step is changing the control flag in the code. When removing control flags, other conditions or variables should be used to control the code's logic. In some cases, the code could be converted into a loop that executes until all desired outcomes are met.
For instance, if isComplete
is the control flag, changing the code could involve implementing logic such that the loop continues to execute until the desired outcomes are achieved, instead of just until isComplete
becomes true.
Step 3: Simplifying the Code
The third and final step involves simplifying the code after removing the control flag. The code should be more straightforward and easier to read. The removal of the control flag should improve the code's readability and eliminate confusion from complex logic flow.
For example, after removing the control flag, the code should be more straightforward and the logic, more apparent. The benefit of this technique is that the code now becomes easier to understand and maintain. However, the technique also has its drawbacks, as it is impossible to have a single approach that works for every scenario. Therefore, one should consider the trade-offs before applying the refactoring technique.
Overall, applying the remove control flag refactoring technique can optimize code and make it more efficient, making it easier to maintain and update. However, it is important to thoroughly understand the code before applying the technique and weigh the benefits and drawbacks.
Summarizing Remove Control Flag Refactoring
The remove control flag refactoring technique is a useful tool for simplifying code and reducing control flags. By following the simple three-step process outlined in this article, you can eliminate unnecessary control flags from your code and make it easier to maintain and read.
However, it is important to be cautious about overusing refactoring techniques, as they can sometimes lead to unnecessary complexity. Keep in mind that the ultimate goal is to create code that is easy to read and maintain, so strive for simplicity whenever possible.
I encourage you to apply the remove control flag refactoring technique in your code to improve its readability and maintainability! By doing so, you'll be able to create more efficient, effective code that can withstand the test of time. Remember to check out my course on Dometrain: Refactoring for C# Devs if you want to skill up on your refactoring! And if you're interested in more learning opportunities, subscribe to my free weekly newsletter and check out my YouTube channel!
Affiliations
These are products & services that I trust, use, and love. I get a kickback if you decide to use my links. There’s no pressure, but I only promote things that I like to use!
- BrandGhost: My social media content and scheduling tool that I use for ALL of my content!
- RackNerd: Cheap VPS hosting options that I love for low-resource usage!
- Contabo: Affordable VPS hosting options!
- ConvertKit: The platform I use for my newsletter!
- SparkLoop: Helps add value to my newsletter!
- Opus Clip: Tool for creating short-form videos!
- Newegg: For all sorts of computer components!
- Bulk Supplements: Huge selection of health supplements!
- Quora: I answer questions when folks request them!