This is an uber-article on all things related to design patterns! Let this be your anchor point to being able to read up on different implementations of design patterns and how you can leverage them.
This article will be "living" because as I add more content to my website for the various design patterns, you'll see more articles referenced here. If you're ever looking to explore more on design patterns, especially how they can be applied in C#, remember to check back!
Remember to get your copy of the E-Book here! All future revisions and additions are FREE!
Remember that you can follow along on these other platforms:
// FIXME: social media icons coming back soon!
What Are Design Patterns
Design patterns are a set of guidelines or templates for solving common design problems in software development. They are proven solutions to recurring design issues and challenges that software developers face during the development of software applications or systems. Here are some key points about design patterns:
-
Reusable Solutions: Design patterns provide standard approaches to solving known problems, which can be reused in different projects and contexts.
-
Best Practices: They are considered best practices that have been evolved over time and are widely accepted among developers. These patterns reflect the experience and insights of professionals and provide an efficient way to tackle common problems.
-
Improve Code Readability: By using design patterns, developers can make their code more understandable and maintainable. Since these patterns are well-known, they provide a common language among developers.
-
Avoid Reinventing the Wheel: Instead of coming up with a new solution every time, design patterns offer time-tested solutions, which helps avoid subtle issues that can cause major problems.
-
Flexibility and Scalability: Some design patterns, like the Strategy or Observer patterns, can make a software system more flexible and scalable. They allow for easier addition of new features or changes in the future.
-
Not a Finished Design: A design pattern is not a finished design that can be directly converted into code. It is a description or template for how to solve a problem that can be used in many different situations.
-
Contextual Usage: Patterns should be applied depending on the context and the specific requirements of the software project. They are not universally applicable for all situations.
By using design patterns, software developers can avoid common pitfalls and write code that is more efficient, scalable, and easy to understand and maintain.
What is the Gang of Four (GoF)?
The "Gang of Four" (GoF) refers to four authors who co-wrote a seminal book on software engineering titled "Design Patterns: Elements of Reusable Object-Oriented Software". This book, first published in 1994, has had a significant impact on software development and is widely regarded as a fundamental resource for understanding and applying design patterns in object-oriented programming. The four authors are:
-
Erich Gamma: He is known for his contributions to software design patterns and software development in general. Gamma has also been involved in the development of the Eclipse platform at IBM.
-
Richard Helm: Helm has expertise in object-oriented programming and is known for his work on design patterns and their application in software development.
-
Ralph Johnson: Johnson is a professor of computer science and an expert in object-oriented programming, especially known for his contribution to the development of design patterns.
-
John Vlissides: He was a software engineer and researcher known for his contribution to design patterns in software engineering. Vlissides passed away in 2005, but his work continues to influence the field.
Their book categorizes design patterns into three types:
- Creational Patterns, for object creation mechanisms.
- Structural Patterns, for composing classes and objects into larger structures.
- Behavioral Patterns, which focus on communication between objects.
The term "Gang of Four" is often used to refer not just to the authors themselves, but also to the list of design patterns they described in their book. These patterns have become a fundamental part of the software engineer's toolkit, providing time-tested solutions to common problems encountered in software design. In this article, you'll see design patterns prefixed with [GoF] to help you identify them.
Creational Design Patterns
Imagine you're building a model airplane. Instead of figuring out each time how to put together the different pieces, you follow a set of instructions or a plan that shows you the best way to do it. In software, a Creational Design Pattern is like this set of instructions. It helps software engineers know the best way to create new objects in their programs. It's like a recipe that tells them how to combine various parts of their code to make a new object that does something specific in their program.
### [GoF] Abstract Factory Design PatternWhat:
- The Abstract Factory Design Pattern is a creational design pattern that provides an interface for creating families of related or dependent objects without specifying their concrete classes.
- It involves a high level of abstraction, typically with multiple factory methods, each responsible for creating different types of objects.
- This pattern is especially useful in systems where the creation process of objects needs to be independent of the main system.
Why:
- To isolate the creation of objects from their usage and to create sets of objects that share a common theme or purpose without depending on their concrete classes.
- Useful when a system should be configured with one of multiple families of products, providing a high level of flexibility.
- Helps in enforcing consistency among products created by a single factory instance.
- Often used in situations where a system needs to be independent of how its products are created, composed, and represented.
How:
- Define an 'Abstract Factory' interface with a set of methods for creating different abstract products.
- Implement the Abstract Factory interface in multiple concrete factory classes, each representing a different variant or family of products.
- Create sets of 'Abstract Product' classes for different kinds of products that are part of the product families.
- Implement concrete product classes for each type of product. These are created by the corresponding concrete factory.
- The client code works with factories and products only through their abstract interfaces. It creates objects through the factory interface, which allows for the creation of products belonging to a specific family.
By applying the Abstract Factory Design Pattern, you can ensure that your code is not bound to the concrete classes of objects that need to be created and is able to support multiple families of products.
[[CAROUSEL tags="design patterns,creational,singleton,factory" max=5]]
[GoF] Builder Design Pattern
What:
- The Builder Design Pattern is a creational design pattern that separates the construction of a complex object from its representation. This allows the same construction process to create different representations.
- It typically involves a 'Builder' interface that defines steps to build a particular complex object, and concrete builder classes that implement these steps for different representations of the object.
- A 'Director' class may be used to manage the construction process using a builder object.
Why:
- To allow the construction of complex objects step-by-step, and to allow the process to be different for different representations.
- Useful when an object needs to be created with many optional components or configurations.
- Helps in improving code readability and maintainability by encapsulating the construction details of complex objects.
- Enables controlling the construction process more finely, as opposed to using numerous constructors or parameter combinations.
How:
- Define a 'Builder' interface with methods for each of the steps required to build a complex object.
- Create concrete 'Builder' classes for each of the different representations of the object, implementing the builder interface.
- Each concrete builder class keeps track of the object it is constructing and provides an interface to retrieve the finished object.
- Optionally, create a 'Director' class that takes a builder object and calls its step methods in a specific order to construct an object.
- The client creates a builder object, passes it to the director (if used), and then retrieves the final object from the builder.
By using the Builder Design Pattern, the process of constructing a complex object is separated from its parts and their assembly, allowing the creation of different representations or configurations of an object from the same construction process.
[GoF] Factory Method Design Pattern
What:
- The Factory Method Design Pattern is a creational design pattern that provides an interface for creating objects in a superclass but allows subclasses to alter the type of objects that will be created. Essentially, it delegates the instantiation of objects to subclasses.
- This pattern involves defining a method in a base class, which is then overridden by subclasses to create specific types of objects.
- It is particularly useful when a class cannot anticipate the class of objects it needs to create or when it wants its subclasses to specify the objects it creates.
Why:
- To provide greater flexibility and encapsulation by allowing class instantiation to be deferred to subclasses.
- Useful in scenarios where a class needs to standardize and centralize the creation process of objects, but the exact types of objects might vary.
- Helps in adhering to the Open/Closed Principle, as new types of objects can be introduced without modifying the existing code.
- Often used when designing frameworks or libraries where implementation details are expected to be extended by client code.
How:
- Define an abstract base class (or interface) with a 'factory method'. This method returns an object of a certain type, like a product.
- Implement the factory method in this class. This method calls the constructor of a product but returns a generic product type.
- Create concrete subclasses of the base class. Each subclass overrides the factory method to return a different type of product.
- The client code calls the factory method to create an object. The actual type of the created object depends on the subclass of the base class that is instantiated.
By using the Factory Method Design Pattern, the process of object creation is isolated from the main code, and the responsibility of which class to instantiate is given to subclasses, leading to a more flexible and scalable code structure.
[GoF] Prototype Design Pattern
What:
- The Prototype Design Pattern is a creational design pattern that allows an object to create a copy of itself. This pattern involves creating new objects by copying a prototype or sample object rather than by creating new instances from scratch.
- The key concept is to use a prototypical instance, which is cloned to produce new objects.
- This pattern is particularly useful when the construction of a new instance is more efficient or convenient by duplicating an existing instance.
Why:
- To avoid the overhead of creating objects in the standard way (using the
new
keyword) when it is more efficient to copy an existing instance. - Useful when an object is required that is similar to an existing object or when the creation process of an object is costly or complex.
- Helps in keeping the system independent of how its objects are created, composed, and represented.
- Often used in scenarios where objects are required that are similar to existing objects, or when objects need to be independent of their creation and representation.
How:
- Define a 'Prototype' interface that declares a method for cloning itself, usually named
clone()
. - Create concrete classes implementing the Prototype interface. These classes implement the
clone()
method to create a copy of themselves. - The Prototype interface allows objects to create copies of themselves without knowing anything about their classes.
- The client, instead of writing code that invokes the
new
operator on a hard-coded class name, calls theclone()
method on a prototype.
By using the Prototype Design Pattern, you can create new objects by copying existing ones, which can be more efficient in certain circumstances, especially when the cost of creating an object is higher due to complexity.
[GoF] Singleton Design Pattern
What:
- The Singleton Design Pattern is a creational design pattern that ensures a class has only one instance and provides a global point of access to it.
- This pattern involves a single class which is responsible for creating its own object while making sure that only a single object gets created. This class provides a way to access its sole object which can be accessed directly without the need to instantiate the object of the class.
Why:
- To control object creation, limiting the number to one but allowing for a flexible number of accessors of that instance.
- Useful in scenarios where exactly one object is needed to coordinate actions across the system, such as in database connections or logging.
- Helps in ensuring that a class has just one instance and provides a global point of access to that instance.
- Often used in scenarios where it's necessary to control shared resources, such as configuration settings, network connections, or hardware interfaces.
How:
- Make the constructor of the class private to prevent other classes from instantiating it.
- Create a private static instance of the class inside the class itself.
- Provide a public static method that returns the instance of the class, this is often called a "getInstance" method. If the instance does not exist, the method creates it; if it does, it returns the existing one.
By using the Singleton Design Pattern, you can ensure that a class only has one instance and provide a global point of access to that instance, which can be particularly useful for managing shared resources or coordinating actions across a system. In our list of design patterns, this one may get the most negative press!
Structural Design Patterns
Think about playing with LEGO blocks. You have different ways to connect these blocks to build something bigger, like a house or a car. In software, a Structural Design Pattern is like these ways of connecting LEGO blocks. It helps software engineers organize different parts of their code so that they work well together, just like how LEGO blocks fit together to create a stable structure.
These patterns are about making sure all the different parts of the program are arranged in a way that makes them strong and efficient when working together. In our list of design patterns, these software design patterns focus on how things are organized.
[GoF] Adapter Design Pattern
What:
- The Adapter Design Pattern is a structural design pattern that allows objects with incompatible interfaces to work together. It acts as a bridge between two incompatible interfaces.
- This pattern involves a single class, known as the adapter, that joins the functionalities of independent or incompatible interfaces. A common scenario where an adapter is used is when new components need to be integrated and work with existing components in the application.
- The adapter translates calls to its interface into calls to the original interface, making it look like a class that the client expects.
Why:
- To enable communication between classes that couldn't otherwise because of incompatible interfaces.
- Useful when integrating new or third-party components with existing components in an application.
- Helps in reusing existing code, as it allows old interfaces to be used with new interfaces.
- Often used when you want to create a reusable class that cooperates with classes that don't have compatible interfaces.
How:
- Define the 'Target' interface that the client uses.
- Create an 'Adapter' class that implements the Target interface.
- The Adapter class has a reference to an instance of the 'Adaptee' class, which has the incompatible interface.
- The Adapter class translates the interface of the Target into the interface of the Adaptee, so the Adaptee's methods can be called by the client through the Target interface.
- The client uses the Adapter class via the Target interface, and the Adapter passes the calls to the methods of the Adaptee.
By applying the Adapter Design Pattern, you can make classes with incompatible interfaces work together, which is essential for reusing existing code or integrating third-party libraries or systems.
[GoF] Bridge Design Pattern
What:
- The Bridge Design Pattern is a structural design pattern that separates an abstraction from its implementation so that the two can vary independently. It involves breaking down a large class or a set of closely related classes into two separate hierarchies—abstraction and implementation—which can be developed independently of each other.
- An 'abstraction' refers to an interface or a layer that the client interacts with, and the 'implementation' refers to the concrete classes that actually implement the abstraction.
- This pattern is useful for avoiding a permanent binding between an abstraction and its implementation and for allowing the coupling between them to be modified at runtime.
Why:
- To avoid a permanent binding between an abstraction and its implementation, allowing them to be independently extended or modified.
- Useful in scenarios where an abstraction and its implementation need to be extended in different ways. For instance, in UI frameworks where the abstraction could be a UI element, and the implementation could be the underlying OS-specific functionality.
- Helps in reducing compile-time dependencies and improving code maintainability and scalability.
- Often used when a class hierarchy should be split into two parts: high-level logical abstraction and low-level platform-specific details.
How:
- Create an 'Abstraction' class that contains a reference to an object of type 'Implementor.'
- Define the 'Implementor' interface that includes the methods that need to be implemented. This interface is not meant to correspond directly to the abstraction interface but to provide basic operations that the abstraction needs.
- Create concrete 'Implementor' classes that provide specific implementations of the Implementor interface.
- The Abstraction class refers to the Implementor but works with all Implementor's concrete classes through the Implementor interface.
- The client interacts with the Abstraction class, and the Abstraction delegates the work to the Implementor object.
By using the Bridge Design Pattern, you can separate an abstraction from its implementation so that both can be changed independently, leading to more flexible and easily maintainable code.
### [GoF] Composite Design PatternWhat:
- The Composite Design Pattern is a structural design pattern that allows you to compose objects into tree structures to represent part-whole hierarchies. This pattern lets clients treat individual objects and compositions of objects uniformly.
- It involves creating a common interface or base class for both individual objects and composite objects. Composite objects can contain other composite objects or leaf objects, but they are treated the same way.
- This pattern is particularly useful for representing hierarchical structures where each element can be either a composite element (containing other elements) or a leaf element (no children).
Why:
- To manage a hierarchy of objects and treat individual and composite objects uniformly.
- Useful in scenarios where clients need to ignore the difference between compositions of objects and individual objects. Clients can treat all objects in the composite structure uniformly.
- Helps to simplify client code, as it can treat composite structures and individual objects the same.
- Often used in graphical applications, file systems, menu systems, and anywhere a tree structure is advantageous.
How:
- Define a 'Component' interface or abstract class with common operations for both simple and composite objects.
- Implement the 'Leaf' class(es) representing simple, indivisible elements. These classes implement the Component interface but typically don't have children.
- Create a 'Composite' class that also implements the Component interface. It stores child components and implements child-related operations in the Component interface.
- The Composite class can add or remove child components (which can be either Leaf or Composite) and implement or delegate its operations to its children.
- The client code interacts with the Component interface to manipulate objects in the composite structure, regardless of their actual concrete type (Leaf or Composite).
By using the Composite Design Pattern, you can create complex tree structures more easily, treating both individual elements and compositions in a uniform manner, which simplifies client code and structure management.
### [GoF] Decorator Design PatternWhat:
- The Decorator Design Pattern is a structural design pattern that allows behavior to be added to individual objects, either statically or dynamically, without affecting the behavior of other objects from the same class.
- This pattern involves a set of decorator classes that are used to wrap concrete components. A decorator class mirrors the type of the component it decorates so that it can be used in place of the original object.
- It's particularly useful when you need to add responsibilities to objects at runtime and you want the capability to add these responsibilities selectively.
Why:
- To extend the functionality of objects without modifying their underlying classes (open/closed principle).
- Useful when subclassing would result in an exponential increase of new classes for every combination of behaviors.
- Helps in adding or modifying responsibilities of objects in a scalable and flexible way.
- Often used in user interface components, stream manipulation, and in situations where it’s beneficial to add features to objects without altering their structure.
How:
- Define a component interface that specifies the standard features.
- Create one or more concrete components that implement this interface.
- Design a decorator class that also implements the component interface and contains a reference to a component object. This decorator class can add additional behaviors (methods) and delegate the component interface's methods to the contained component object.
- Create concrete decorator classes that extend the base decorator class. Each of these classes adds its specific functionality either before or after calling the super-class method (which would call the component method).
- The client code can then use these decorators to add functionalities to the base component dynamically.
By using the Decorator Design Pattern, you can dynamically add or modify the behavior of individual objects without affecting other objects from the same class, providing a flexible alternative to subclassing for extending functionality.
### [GoF] Facade Design PatternWhat:
- The Facade Design Pattern is a structural design pattern that provides a simplified interface to a complex system of classes, library, or framework. It's like a "front-facing" interface masking more complex underlying or structural code.
- The facade doesn't encapsulate the complex subsystem; instead, it provides a simplified interface to the complex parts of the subsystem.
- It's particularly useful when a system is very complex or difficult to understand because the system has a large number of interdependent classes or its source code is unavailable.
Why:
- To make a software library easier to use, understand, and test, and to reduce dependencies on external code.
- Useful for dividing a system into subsystems to reduce complexities and to minimize the communication and dependencies between subsystems.
- Helps in isolating clients from the complexities of the subsystem components, thereby reducing the number of objects that clients deal with and making the subsystem easier to use.
- Often used in situations where there is a need for an easy-to-use interface to a complex framework or a large body of code.
How:
- Create a 'Facade' class that provides a simple interface to the complex functionality of one or more subsystems. This class delegates the client's requests to the appropriate objects within the subsystem.
- The facade should know which subsystem classes are responsible for a request and delegate the requests to the appropriate objects.
- Clients communicate with the subsystem by sending requests to the Facade, which then passes them to the appropriate subsystem objects.
- The subsystem itself remains fully functional and can be used independently of the facade, but the facade simplifies the subsystem usage for common tasks.
By using the Facade Design Pattern, you can provide a simple interface to a complex system, making it easier for other systems or clients to interact with it without understanding the complexities beneath.
### [GoF] Flyweight Design PatternWhat:
- The Flyweight Design Pattern is a structural design pattern that aims to minimize memory usage or computational expenses by sharing as much as possible with similar objects. It's about sharing to support large numbers of fine-grained objects efficiently.
- In this pattern, a flyweight is a shared object that can be used in multiple contexts simultaneously, appearing as an independent object in each context. It's particularly useful when a program requires a large number of objects that have similar state.
- The pattern involves separating the intrinsic state (internal state that is shared) from the extrinsic state (external state that is specific to the context).
Why:
- To reduce the number of objects needed and the memory footprint of an application, especially when a simple repeated representation would consume an excessive amount of memory.
- Useful in scenarios where a large number of similar objects are needed, as it can significantly reduce the resource load by sharing.
- Enhances performance in applications with a large number of similar objects, like graphical applications or games.
- Can help in improving application performance and memory usage significantly.
How:
- Create a Flyweight interface that declares methods which flyweight instances can receive and use extrinsic data.
- Create one or more concrete Flyweight classes that implement this interface. The concrete Flyweight stores intrinsic state that is common and shareable.
- The Flyweight Factory manages the flyweight objects. It creates and manages the flyweights, ensuring that flyweights are shared properly. When a client requests a flyweight, the factory either returns an existing instance or creates a new one if none exists.
- The client must store or compute the extrinsic state (context-specific state) and pass it to the flyweight methods.
By applying the Flyweight Design Pattern, you can efficiently handle a large number of objects by sharing common parts among them, thereby reducing memory consumption and potential processing overhead in your application.
### Plugin Design PatternWhat:
- The Plugin Design Pattern allows for the extension or alteration of an application's functionality by adding or removing independent modules, known as plugins.
- It involves a core system that defines a standard interface for plugins, and plugins implement this interface to interact with the core system.
- This design pattern separates the core functionality from the extended features, promoting modularity and flexibility.
Why:
- Extensibility: Enables adding new features or modifying existing ones without altering the core application code.
- Flexibility: Allows for the customization of applications to meet different requirements or preferences.
- Maintainability: Simplifies updates and maintenance since plugins can be modified independently of the core system.
- Modularity: Encourages a modular architecture, making the system more organized and easier to manage.
How:
- Define a Plugin Interface: The core system defines an interface or a set of hooks that establish how plugins can interact with it.
- Develop Plugins: Create plugins as separate modules or components that implement the defined interface.
- Integration: The core application detects, loads, and integrates plugins, often at runtime, allowing them to extend or modify the application’s capabilities.
- Plugin Management: Optionally, include a plugin manager in the core system to handle the loading, unloading, and managing of the plugins.
This approach is language-agnostic and can be implemented in various programming environments to create systems that are capable of evolving and adapting through the integration of plugins.
### [GoF] Proxy Design PatternWhat:
- The Proxy Design Pattern is a structural design pattern that involves using a placeholder or substitute object (the proxy) to represent another object (the real subject).
- This pattern is used to control access to the real subject, which might be remote, expensive to create, or in need of securing.
- The proxy and the real subject usually share a common interface, allowing the proxy to stand in for the real subject.
Why:
- To provide a surrogate or placeholder for another object to control access to it. This is useful in various scenarios like lazy initialization, access control, logging, or handling expensive operations.
- Particularly beneficial when the real subject is remote (like in network operations), large and resource-intensive, or sensitive in terms of security.
- Helps in adding a level of indirection to the real subject, which can protect it or optimize its operations without altering its code.
- Can also be used for caching purposes, delaying the instantiation of large objects, or implementing reference counting.
How:
- Define a 'Subject' interface that declares common operations for both the real subject and the proxy.
- Implement the real subject class that does the actual work.
- Create a proxy class that also implements the Subject interface. This class maintains a reference to the real subject.
- The proxy intercepts all calls to the real subject and can add its behavior (like initializing the real subject, checking permissions, or adding caching) before or after delegating the calls to the real subject.
- The client interacts with the proxy as if it were the real subject, unaware of the proxy’s existence.
By using the Proxy Design Pattern, you can control interactions with an object, add additional behaviors, and manage its resource consumption more efficiently, all without altering the object itself.
## Behavioral Design Patterns
Imagine you're on a sports team, like a soccer team. Each player has a role, like a goalkeeper or a striker, and they have to work together following certain strategies to win the game. In software, a Behavioral Design Pattern is like these strategies on a sports team. It helps software engineers manage how different parts of their program communicate and work together. It's about coordinating the actions and interactions of different parts of the program, like how players on a soccer team coordinate their movements and actions to play the game effectively.
### [GoF] Chain of Responsibility Design PatternWhat:
- The Chain of Responsibility Design Pattern is a behavioral design pattern that allows an object to send a command or a request along a chain of potential handlers until one of them handles the request.
- It involves a source of command objects and a series of processing objects. Each processing object in the chain is responsible for a specific type of command and decides either to process the command or to pass it to the next object in the chain.
- This pattern is useful for decoupling a request's sender and its receivers by giving multiple objects a chance to handle the request.
Why:
- To reduce the coupling between the sender of a request and its receivers. The sender only knows that the request will be handled appropriately, not by which exact handler.
- Useful in scenarios where more than one object may handle a request, and the handler is not known a priori. The request can be passed along the chain until a handler is found.
- Enhances flexibility in assigning responsibilities to objects. Handlers can be added or changed dynamically without affecting the sender.
- Often used in event handling systems, logging operations, and processing pipelines.
How:
- Define a 'Handler' interface specifying a method for handling requests and optionally implementing a successor link (the next handler in the chain).
- Create concrete handler classes that implement the Handler interface. Each handler decides whether to process the incoming request and/or pass it to the next handler in the chain.
- Link the handlers together to form a chain, where each handler has a reference to the next handler.
- The client sends requests to the first handler in the chain. The request travels along the chain until a handler handles it, or it reaches the end of the chain unhandled.
By implementing the Chain of Responsibility Design Pattern, you enable the dynamic handling of a set of requests, each by a different handler, in a flexible and extendable manner. This leads to a more decoupled system where senders and receivers of requests are less tightly bound.
### [GoF] Command Design PatternWhat:
- The Command Design Pattern is a behavioral design pattern that turns a request or simple operation into an object. This pattern involves encapsulating all the information needed to perform an action or trigger an event at a later time.
- It typically includes the command itself, its parameters, and any other necessary information.
- This pattern is used to decouple objects that send a request from the objects that receive and execute them.
Why:
- To separate the responsibility of issuing commands from the responsibility of executing commands. This separation allows for more flexible command processing and easier command management.
- Useful in scenarios where commands need to be queued, undone, or logged.
- Enhances flexibility and reusability as new commands can be added without changing existing code.
- Often used in graphical user interfaces, transactional systems, and other scenarios where actions need to be triggered and possibly undone.
How:
- Define a 'Command' interface with a method for executing the command.
- Create one or more concrete classes implementing the Command interface. Each class represents a specific command and encapsulates the action to be executed.
- The 'Invoker' class takes and stores commands. It can issue these commands without knowing anything about the operation's specifics or the receiver of the command.
- The 'Receiver' class knows how to perform the operations associated with carrying out a request. Any class can act as a Receiver.
- The client creates command objects and associates them with receivers. These commands are then passed to an invoker object which executes them.
By applying the Command Design Pattern, you can parameterize objects with operations, delay or queue a command's execution, and support undoable operations, thereby adding significant flexibility to your software.
### [GoF] Interpreter Design PatternWhat:
- The Interpreter Design Pattern is a behavioral design pattern that is used for defining a grammatical representation for a language and providing an interpreter to deal with this grammar.
- Essentially, it's about defining a language's grammar in a way that a program can understand and then creating an interpreter that can process and execute commands written in that language.
- The pattern is commonly used in compilers, parsers, and expression processors where interpreting a language or an expression according to a defined grammar is necessary.
Why:
- To provide a way to interpret sentences in a language or expressions within a program.
- Useful when a particular type of problem involves processing language-based instructions, like mathematical expressions, SQL queries, or communication protocols.
- It helps in separating the expression’s interpretation process from the expressions themselves, making the code more modular and easier to extend or modify.
- Allows for dynamic interpretation of expressions at runtime, which is useful in scripting, programming languages, or complex query processing.
How:
- Define an 'Expression' interface with a method for interpreting a context (the text or language to be interpreted).
- Implement the Expression interface in concrete classes for each rule in the grammar. These classes represent various grammar rules or expressions.
- The context class contains information that's global to the interpreter, usually the specific language or text being interpreted.
- The client creates an abstract syntax tree representing a particular sentence in the language the grammar defines, using instances of the expression classes.
- The tree is then traversed, and each node is interpreted in the context of the interpretation.
By implementing the Interpreter Design Pattern, software engineers can efficiently process and interpret language or expression-based instructions within their applications in a structured and scalable manner.
### [GoF] Iterator Design PatternWhat:
- The Iterator Design Pattern is a behavioral design pattern that provides a way to access the elements of an aggregate object sequentially without exposing its underlying representation.
- It involves two key components: the iterator, which is responsible for accessing and traversing elements, and the collection, which is the aggregate object being iterated over.
- This pattern is widely used in software development to traverse various types of collections, such as lists, trees, and graphs, in a standardized way.
Why:
- To provide a uniform way to traverse different data structures without exposing the internal details of these structures.
- Useful for accessing a collection's elements without understanding or handling its complex underlying structure.
- Helps in keeping the iteration logic separate from the collection, leading to cleaner, more maintainable code.
- Enables the implementation of different types of iterators that can provide different traversal strategies over the same collection.
How:
- Define an 'Iterator' interface with methods for operations like accessing the next element, checking if there are more elements, and possibly removing an element.
- Implement the Iterator interface in one or more concrete iterator classes, each providing the logic for traversing a specific kind of data structure.
- The collection (or aggregate) class provides a method to create and return an instance of the appropriate iterator class. This method is often named something like
createIterator()
. - The client, which needs to traverse the elements of the collection, uses the iterator's interface, allowing it to navigate through the collection without requiring detailed knowledge of its internal structure.
By applying the Iterator Design Pattern, you enable efficient traversal of different data structures in a consistent way, which decouples the algorithms from the data structures they work on.
### [GoF] Mediator Design PatternWhat:
- The Mediator Design Pattern is a behavioral design pattern that provides a centralized communication medium between different objects in a system.
- Instead of objects communicating directly with each other and thus being tightly coupled, they interact through a mediator object. This mediator handles the interactions and routing of messages or data between them.
- The pattern is useful in complex systems where the interaction between objects is extensive or complicated.
Why:
- To reduce direct communication between objects, thereby decreasing the coupling between them, which makes the system easier to understand and maintain.
- It simplifies the management of interactions and makes it easier to change the interaction logic independently.
- Particularly helpful in GUI applications or systems where multiple components need to interact, but direct communication would lead to a tangled web of interactions.
- Helps in keeping each object's focus on its core functionality while the mediator handles the communication logic.
How:
- Create a 'Mediator' interface that defines the methods for communicating with the objects (colleagues).
- Implement the Mediator interface in a concrete mediator class. This class coordinates the interaction between the colleague objects.
- Define a set of 'Colleague' classes that interact with each other through the mediator. These colleagues know only about the mediator and not about each other.
- Colleagues use the mediator to send messages to other colleagues, and the mediator then routes these messages to the appropriate colleague(s).
- When the state of a colleague changes in a way that requires communication with other colleagues, it sends a notification to the mediator instead of communicating directly with other colleagues.
By using the Mediator Design Pattern, you can centralize complex communications and control mechanisms between various components, leading to a design that's easier to understand, maintain, and extend.
### [GoF] Memento Design PatternWhat:
- The Memento Design Pattern is a behavioral design pattern that allows capturing and externalizing an object's internal state so that the object can be restored to this state later.
- It involves three key elements: the originator (the object whose state needs to be saved), the memento (the object that keeps the saved state), and the caretaker (which keeps track of the memento).
- This pattern is particularly useful for implementing undo functionalities or for taking snapshots of an object's state which can be restored later without exposing the object's internal structure.
Why:
- To provide a mechanism to restore an object to its previous state without revealing the details of its implementation.
- Useful in scenarios where direct access to the object's internal state is not desirable or violates encapsulation principles.
- Helps in keeping the originator's state encapsulation intact while still allowing state preservation and restoration.
- Particularly beneficial in applications like text editors, games, or other software where users might need to undo actions or restore previous states.
How:
- Create a 'Memento' class that stores the internal state of the 'Originator' object. The Memento should have two interfaces: one for the caretaker, which allows storing and retrieving the memento, and one for the originator, which allows reading and writing the internal state.
- Implement the 'Originator' class, which includes methods for saving its state to a memento and restoring its state from a memento.
- Implement a 'Caretaker' class that keeps track of the mementos, but without examining or operating on their contents.
- When a state needs to be saved, the caretaker asks the originator to create a memento and stores it. When a state restore is needed, the caretaker gives the memento back to the originator for state restoration.
By applying the Memento Design Pattern, you can effectively manage snapshots of an object's state for easy retrieval and restoration, while maintaining the principles of encapsulation and internal state protection.
### [GoF] Observer Design PatternWhat:
- The Observer Design Pattern is a behavioral design pattern where an object, known as the subject, maintains a list of its dependents, called observers, and notifies them automatically of any state changes.
- This pattern is used to establish a one-to-many dependency between objects so that when one object changes its state, all its dependents are notified and updated automatically.
- It's commonly used for implementing distributed event handling systems and in situations where one object needs to notify others about changes in its state.
Why:
- To achieve low coupling between the subject and its observers. The subject doesn't need to know anything about the observers, just that they implement a certain interface.
- Useful for scenarios where a change to one object requires changing others, and the number of objects that need changing is unknown or dynamic.
- Enhances flexibility and reusability as new observers can be added without modifying the subject.
- Often used in graphical user interfaces and data monitoring scenarios.
How:
- Create a 'Subject' interface that defines methods for attaching, detaching, and notifying observers.
- Implement the Subject interface in a concrete class. This class maintains a list of observers and notifies them of any state changes.
- Create an 'Observer' interface with a method for receiving updates from the subject.
- Implement the Observer interface in concrete classes that define how they should be updated.
- The subject class calls the update method of all its observers when its state changes, ensuring that they are aware of the new state.
By using the Observer Design Pattern, you can ensure that changes in one object's state are automatically propagated to all interested objects without needing to maintain a hard-coded list of dependencies.
### Pipeline Design PatternWhat:
- The Pipeline Design Pattern is a structural design pattern that helps manage a sequence of processing steps or a pipeline of operations.
- It allows for organizing complex processing steps into a set of simpler, reusable components (stages).
- This pattern is ideal for scenarios where data or tasks need to be processed in distinct, logical stages.
Why:
- To streamline and organize complex processing logic.
- Enhances code readability and maintainability by breaking down a process into manageable parts.
- Facilitates reusability of processing steps and allows for flexible modification of the processing sequence.
- Useful in scenarios like data processing, request handling, or workflow management.
How:
- Define an interface for each stage in the pipeline, specifying the input and output data types.
- Implement each stage as a separate component following the defined interface.
- Chain the stages together, where the output of one stage becomes the input for the next.
- Optionally, add functionality for error handling and data transformation between stages.
By applying the Pipeline Design Pattern, developers can create modular, efficient, and manageable workflows, simplifying the process of handling complex data transformations and operations.
### Specification Design PatternWhat:
- The Specification Design Pattern is a structural design pattern that allows the encapsulation of business rules or criteria.
- It separates the statement of how to match a candidate, from the candidate object that it is matched against.
- This pattern is helpful for building a system where business rules are changeable, complex, and need to be combined in various ways.
Why:
- To create a flexible system that handles complex, changeable business rules.
- Reduces duplication of decision logic across the application.
- Allows business rules to be composed using boolean logic.
- Enhances testability and separation of concerns by isolating business rules in separate classes.
How:
- Define a 'Specification' interface with a method that determines if a candidate meets a particular criterion.
- Implement concrete specification classes for each specific business rule.
- Use these specifications in the application to filter, select, or validate objects.
- Combine specifications using logical operations (like AND, OR, NOT) to create more complex rules.
By applying the Specification Design Pattern, developers can create a system with complex business rules that are easy to manage, extend, and combine in flexible ways.
### [GoF] State Design PatternWhat:
- The State Design Pattern is a behavioral design pattern that allows an object to change its behavior when its internal state changes.
- It encapsulates the varying behavior for each state within state-specific classes.
- This pattern is useful for objects that need to change their behavior dynamically depending on internal conditions or state.
Why:
- To manage state-related behavior and transitions in a clean and maintainable way.
- Avoids excessive use of conditional statements (like if-else or switch-case) in objects that have different behavior based on their state.
- Makes state transitions explicit and more manageable by representing each state with a separate class.
- Helps in organizing the code better and makes it more extensible, as new states can be added with minimal changes to existing code.
How:
- Define a 'State' interface outlining the methods that represent the behavior associated with a particular state of the object.
- Implement different state classes, each representing a specific state of the object, and implement the methods defined in the 'State' interface.
- The context class (the class whose behavior varies) contains a reference to a 'State' object that represents its current state.
- The context delegates the behavior to the 'State' object.
- As the state of the context changes, it switches its 'State' object to a different subclass, thus changing its behavior dynamically.
By applying the State Design Pattern, objects can change their behavior dynamically by changing their internal 'State' objects, leading to cleaner and more manageable code.
### [GoF] Strategy Design PatternWhat:
- The Strategy Design Pattern is a behavioral design pattern that enables selecting an algorithm's behavior at runtime.
- It defines a family of algorithms, encapsulates each one, and makes them interchangeable within that family.
- The pattern involves defining a strategy interface for a set of behaviors and implementing this interface in various concrete strategy classes.
Why:
- To allow the switching of algorithms or strategies under certain circumstances within an application.
- Useful when there are multiple ways of performing a task and the best approach depends on the context.
- Helps in avoiding conditional statements for selecting desired behavior, leading to more maintainable, modular, and testable code.
- Supports the Open/Closed Principle by allowing the extension of the system with new strategies without modifying existing code.
How:
- Define a strategy interface common to all supported algorithms. This interface declares a method the context will use to call the algorithm.
- Create concrete classes implementing the strategy interface, each representing a different algorithm.
- Define a context class that contains a reference to a strategy object. This context delegates the execution of the algorithm to the linked strategy object.
- The context class is configured with a concrete strategy object at runtime, and it exposes a method to the outside world to allow clients to change the strategy.
- Clients of the context class interact with it through its interface, and the context utilizes the strategy objects to perform the work.
By applying the Strategy Design Pattern, you can define a set of algorithms, encapsulate each one, and make them interchangeable to vary the behavior of an application during runtime.
### [GoF] Template Method Design PatternWhat:
- The Template Method Design Pattern is a behavioral design pattern that defines the program skeleton of an algorithm in a method, deferring some steps to subclasses.
- It allows subclasses to redefine certain steps of an algorithm without changing the algorithm's structure.
- The pattern is based on inheritance: it uses abstract classes and inheritance to allow a template method to call abstract methods, which are implemented by subclasses.
Why:
- To enable the customization of parts of an algorithm without altering its structure.
- Useful when multiple classes do something similar but with slight differences. The common parts of the algorithm can be centralized to avoid code duplication.
- It promotes reusability and inversion of control, as subclass-specific behavior is defined by subclasses themselves, not the parent class.
- Helps in enforcing a standard algorithm structure, ensuring that certain steps are executed in a particular order.
How:
- Create an abstract base class that defines the template method. This method outlines the skeleton of an algorithm, composed of a series of steps, some of which are abstract.
- Implement the invariant parts of the algorithm directly within the template method.
- Define abstract methods (or hooks) for the variant steps, which will be implemented by subclasses.
- Subclasses override these abstract methods to provide concrete behavior but do not override the template method itself.
- When the template method is called on an instance of a subclass, it executes the algorithm, calling the subclass's implementations of the abstract methods.
By using the Template Method Design Pattern, you create a framework that guides the execution of an algorithm, allowing subclasses to provide specific behavior in a controlled manner.
### [GoF] Visitor Design PatternWhat:
- The Visitor Design Pattern is a way of separating an algorithm from the objects it operates on.
- It involves creating a visitor class that implements various operations to be performed on elements of different classes.
- The pattern allows adding new operations without modifying the classes of the elements on which it operates.
Why:
- To add new operations or functionalities to a set of objects without changing the classes of these objects.
- To concentrate related operations in a single class rather than spread across multiple classes.
- Useful in situations where a set of classes varies frequently, but the operations performed on them are relatively stable.
- Helps in maintaining Open/Closed Principle, as it enables classes to be open for extension but closed for modification.
How:
- Define a 'Visitor' interface with a visit method for each type of element in the object structure.
- Implement the Visitor interface in a concrete visitor class for each different operation.
- Each element class in the object structure must have an accept method that takes a visitor as an argument.
- The accept method of an element calls the visit method of the visitor, passing itself as an argument.
- This allows the visitor to perform the operation on the element, and different visitors can perform different operations.
By using the Visitor Design Pattern, you can easily add new operations on a set of objects without altering the classes of those objects, making your code more maintainable and flexible.
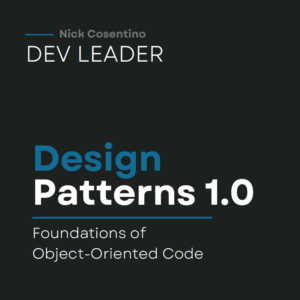