In this article, I’ll be answering for you “What is string interpolation in C#” — which is probably why you arrived here! As a C# developer, it is essential to write readable code. One great tool we have to help us with this is string interpolation in C#. Dotnet string interpolation is the process of combining variables and string literals into a single string, without the awkward placeholder and formatter pattern. The result is more readable and maintainable code that executes faster than traditional string concatenation.
Creating readable and expressive code should be a priority for software engineers. With dotnet string interpolation, we can achieve both efficiency and readability in our code. In this article, we’ll explore what string interpolation is, its benefits, and how to use it in C#. By the end of this article, you’ll gain a better understanding of this powerful feature and learn how to write more optimized and efficient code in C#.
Let’s dive in!
Exploring: What Is String Interpolation In C#
- What is String Interpolation in C#
- Benefits of Using String Interpolation in C#
- BenchmarkDotNet Comparisons for String Interpolation in C#
- Common Mistakes to Avoid
- DotNet String Interpolation Best Practices
- Wrapping Up String Interpolation in C#
- Frequently Asked Questions – What Is String Interpolation In C#
- What is String Interpolation in C#?
- How does String Interpolation differ from String Concatenation?
- What are the benefits of using String Interpolation in C#?
- How does String Interpolation improve code readability?
- How does String Interpolation increase efficiency?
- How do I use String Interpolation in C#?
- What are some common mistakes to avoid when using String Interpolation?
- What are the best practices for using String Interpolation in C#?
What is String Interpolation in C#
String interpolation is a feature in C# that allows developers to embed expressions inside string literals. This means that variables, functions or expressions can be included within a string, without needing to concatenate strings together using the ‘+’ operator. The syntax for using string interpolation in C# is simple. To embed an expression within a string, use the ‘$’ symbol before the opening double-quote, followed by the expressions enclosed in curly brackets ‘{}’.
String Concatenation in C#
In contrast, string concatenation is a way of building a new string by combining multiple strings together using the ‘+’ operator. This can become cumbersome and difficult to read, especially with long strings and multiple string concatenations.
To show the difference between string concatenation and string interpolation, let’s take a look at an example:
string name = "John";
// String concatenation example
string greeting = "Hello " + name + ", welcome to our website!";
// String interpolation example
string greeting = $"Hello {name}, welcome to our website!";
As you can see, the string interpolation example is much easier to read and write, as it allows developers to directly insert variables into a string.
String.Format in C#
An alternative that we had access to before string interpolation in C# is using String.Format. This accomplishes functionally the same outcome, but the readability is more difficult. This is because as readers, we need to mentally do the replacement in order to see where the values will be placed.
Let’s look at a similar example with String.Format:
string first = "John";
string last = "Smith";
// String format example
string greeting = string.Format(
"Hello {0} {1}, welcome to our website!",
first,
last);
// String interpolation example
string greeting = $"Hello {first} {last}, welcome to our website!";
Even with this simple example, we can see that a small amount of indirection to do the value replacement mentally is less readable. While it seems trivial, this kind of thing adds up in cognitive load — especially with more complex strings.
Benefits of Using String Interpolation in C#
Using string interpolation in C# has many benefits for developers, which can help to greatly improve code readability and efficiency.
Improved Readability
One of the primary benefits of using string interpolation is the improved readability of the code. When variables or expressions are embedded within a string using string interpolation, it becomes much easier to read and understand the intent of the code.
For example, let’s take a look at the following code:
// String concatenation example
string firstName = "John";
string lastName = "Doe";
string greeting = "Hello " + firstName + " " + lastName + ", welcome to our website!";
This code can become confusing, especially with longer strings and more complex concatenation. Instead, string interpolation provides an easier solution:
// String interpolation example
string firstName = "John";
string lastName = "Doe";
string greeting = $"Hello {firstName} {lastName}, welcome to our website!";
In summary, string interpolation can greatly improve the readability of code by making it easier to include variables and expressions within a string.
Increased Efficiency
Another major benefit of using string interpolation is the increase in efficiency when compared to string concatenation. When multiple strings are concatenated together, a new string object is created in memory for each concatenation, resulting in a slower performance. On the other hand, with string interpolation a single string is created directly in memory which is faster than string concatenation. This can provide significant performance improvements for applications that require numerous string concatenations.
But don’t just take my word for it, let’s check out some benchmarks in BenchmarkDotNet!
BenchmarkDotNet Comparisons for String Interpolation in C#
We’re going to be comparing string interpolation in C# primarily with string concatenation and string format. These other two are the common ways that people will format strings inside of their C# programs, but using a StringBuilder is also a viable option. I’ll leave the StringBuilder comparisons for another write up.
The Benchmark Setup Code
Let’s check the following BenchmarkDotNet code:
using System.Reflection;
using BenchmarkDotNet.Attributes;
using BenchmarkDotNet.Order;
using BenchmarkDotNet.Running;
BenchmarkSwitcher switcher = new(Assembly.GetExecutingAssembly());
switcher.Run(args);
[ShortRunJob]
[MemoryDiagnoser]
[Orderer(SummaryOrderPolicy.Method, MethodOrderPolicy.Alphabetical)]
public class Benchmarks
{
private const string ConstantWord = "World";
private readonly string _parameter;
// world is 5 characters long
[Params(5, 100, 1_000, 10_000)]
public int ParamerterLength;
public Benchmarks()
{
_parameter = new string('a', ParamerterLength);
}
[Benchmark]
public void Constant_StringFormat()
{
var xxx = string.Format("Hello {0}!", ConstantWord);
}
[Benchmark]
public void Constant_StringInterpolation()
{
var xxx = $"Hello {ConstantWord}!";
}
[Benchmark]
public void Constant_StringConcatenation()
{
var xxx = "Hello " + ConstantWord + "!";
}
[Benchmark]
public void Variable_StringFormat()
{
var xxx = string.Format("Hello {0}!", _parameter);
}
[Benchmark]
public void Variable_StringInterpolation()
{
var xxx = $"Hello {_parameter}!";
}
[Benchmark]
public void Variable_StringConcatenation()
{
var xxx = "Hello " + _parameter + "!";
}
[Benchmark]
public void Variable10N_StringInterpolation()
{
var xxx = $"{_parameter}{_parameter}{_parameter}{_parameter}{_parameter}{_parameter}{_parameter}{_parameter}{_parameter}{_parameter}";
}
[Benchmark]
public void Variable10N_StringConcatenation()
{
var xxx = _parameter + _parameter + _parameter + _parameter + _parameter + _parameter + _parameter + _parameter + _parameter + _parameter;
}
}
The variations that I’m including in the code are:
- String Format
- String Concatenation
- String Interpolation
But I’m also doing two flavors of the above:
- Constant Value: At compile time, the compiler *should* know the full string that will result from formatting.
- Variable Value: We need to wait until runtime to be able to to know the full string that will result from formatting.
I’m doing this because I want to highlight allocation and performance. Theoretically, we should get the best performance when dealing with constants, but let’s see what the results are.
BenchmarkDotNet Results
Let’s have a look at the results below. Remember, to click the picture for a higher resolution look!
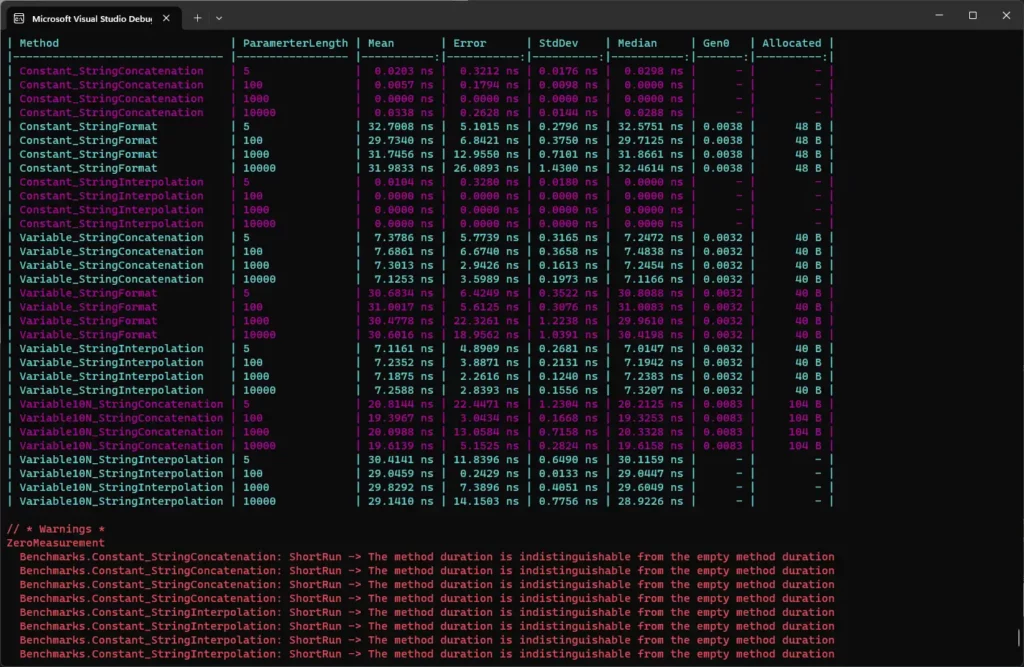
The first thing to notice: String.Format() does worse for performance and memory usage across the board here. For me personally, I only use String.Format if I’m doing some data-templating where the template of the string I’m formatting is external. This is pretty rare for me though.
Constant string concatenation and string interpolation both have zero allocation and effectively instantaneous performance. You can see the warning at the bottom that tells us that BenchmarkDotNet can’t tell the difference between this and an empty method. However, the variable forms of these look VERY comparable.
I decided to include the last two scenarios with “10N” in the name because there’s 10 instances of interpolation or concatenation. The interpolated strings were slightly worse for performance but they were zero allocation. This becomes interesting because we’d be trading memory allocation vs a slight performance gain. But I think that a StringBuilder would be an interesting follow up for this!
Common Mistakes to Avoid
When using string interpolation in C#, there are some common mistakes that can be made. Here are a few tips to help you avoid these mistakes:
- When using curly brackets within a string literal, be sure to use the correct syntax (i.e. ‘{‘ and ‘}’).
- Be sure to include the ‘$’ symbol before the opening double-quote to indicate that the string is being interpolated.
- Double-check that your expressions within the curly brackets are valid and can be evaluated.
- If your expression inside of your curly braces is not compiling, try wrapping that expression inside of parentheses as well.
By following these tips and using string interpolation in C# correctly, you can greatly improve code readability and efficiency.
DotNet String Interpolation Best Practices
As with any coding technique, there are certain best practices to follow when using string interpolation in your C# code. In this section, we’ll cover some tips and recommendations for when to use string interpolation, situations where string concatenation may be better, and best practices for keeping your code organized and readable.
Recommendations for When to Use DotNet String Interpolation
String interpolation is a great tool for simplifying and improving the readability of your code. However, there are certain situations where it is more appropriate to use than others.
String interpolation is best used when:
- You need to concatenate multiple strings together (that aren’t huge) to create a complex output. A StringBuilder may be a better option in some situations.
- You want to embed variables or expressions directly into a string.
- You want to improve the readability of your code.
Situations Where String Concatenation May Be Better
Despite its usefulness, string interpolation may not always be the best choice. For example, you may want to use string concatenation instead of string interpolation when:
- You are working with dynamic string building or conditional string concatenation.
- You are dealing with complex formatting or multi-line strings. Use your discretion if it’s making it difficult to understand the format.
- You are working with very large strings, and don’t want to risk the performance penalties associated with string interpolation where a StringBuilder may be more effective.
Best Practices for Keeping Code Organized and Readable
To keep your code organized and readable when using string interpolation, there are a few best practices you can follow:
- Keep your interpolated string short and readable.
- Use descriptive variable names, and avoid using abbreviations or acronyms.
- Use the same style and naming conventions throughout your code.
- Prefer string interpolation over concatenation, but don’t overuse it.
By following these best practices, you can use string interpolation effectively and improve the maintenance and readability of your code.
Wrapping Up String Interpolation in C#
So what is string interpolation in C#? It’s a super convenient tool that every C# developer should know how to use. By optimizing your code with string interpolation, you can greatly improve the readability and efficiency of your code. Some of the benefits of using string interpolation include streamlined code, improved readability, and increased efficiency.
It is important to keep in mind that string interpolation is not always the best choice for every situation. You should carefully evaluate your options and determine whether string interpolation or string concatenation is best for your specific code. Remember the benchmarks that we looked at? And remember, those don’t include StringBuilder yet either!
In order to become a better C# developer, it is important to continue learning and practicing. If you’re interested in more learning opportunities, subscribe to my free weekly newsletter and check out my YouTube channel!
Frequently Asked Questions – What Is String Interpolation In C#
What is String Interpolation in C#?
String interpolation is a way to embed expressions into string literals using placeholders, denoted by a dollar sign followed by curly braces. This allows for more efficient and readable code compared to using string concatenation to combine multiple strings and variables.
How does String Interpolation differ from String Concatenation?
String interpolation and string concatenation are both ways of combining strings and variables, but interpolation simplifies the process by allowing variables to be embedded directly into the string without the need for additional operators or syntax. This results in cleaner, more efficient code.
What are the benefits of using String Interpolation in C#?
Using string interpolation in C# can result in improved code readability and increased efficiency compared to using string concatenation. Code is easier to read and understand with embedded placeholders, and performance is improved since interpolation avoids the overhead of creating intermediate strings when concatenating.
How does String Interpolation improve code readability?
String interpolation makes code easier to read by allowing placeholders for expressions, variables or literals to be directly embedded into the string. This avoids the need for additional operators or syntax required with string concatenation, resulting in clearer, more understandable code.
How does String Interpolation increase efficiency?
String interpolation can be faster than string concatenation due to the fact that intermediary strings are not created during concatenation. This results in improved performance and efficiency of the code.
How do I use String Interpolation in C#?
To use string interpolation in C#, simply use a dollar sign followed by curly braces to denote the placeholders for expressions, variables or literals.
What are some common mistakes to avoid when using String Interpolation?
Some common mistakes to avoid when using string interpolation include forgetting to use the dollar sign followed by curly braces to denote placeholders, not properly formatting expressions or variables within the placeholders, and not properly escaping characters within the string literal.
What are the best practices for using String Interpolation in C#?
Best practices for using string interpolation in C# include using it for simple string concatenation tasks, avoiding its use for complex or lengthy string building, and keeping the code organized and readable by properly formatting expressions and variables within the string.