Refactoring is an essential practice in software development, involving modifying existing code without changing its external behavior. And while that's the typical goal, I'd make the argument it's what we strive towards because we can certainly refactor and plan to have behavior changes. Refactoring allows for improvements in the codebase's readability, maintainability, performance, and chasing down tech debt. In this article, I'll share a list of top 10 refactoring techniques to help you transform your codebase.
As software engineers or aspiring software engineers, I think that it's critical to be familiar with these techniques to work more efficiently and effectively. Throughout this article, I'll provide examples of refactoring using the C# programming language. I hope this article will be useful in helping you transform your codebase towards best practices, so let's dive in!
Understanding Refactoring
Refactoring is the process of modifying code to enhance its structure, design, and maintainability without altering its external behavior. It's a fundamental aspect of software development that is necessary to eliminate technical debt and make code more adaptable and scalable. Refactoring allows codebases to evolve without sacrificing quality and efficiency.
It's important to distinguish refactoring from rewriting code, which is the process of writing new code from scratch. Code rewriting is often an extreme measure that involves throwing away existing code and starting from scratch. In contrast, refactoring seeks to improve existing code without tossing it away. Code rewriting is often done as a last resort whereas refactoring should be done throughout the development cycle.
Refactoring code becomes necessary for several reasons. One of the reasons is to eliminate technical debt, which is the cost of maintaining code that deviates from clean code practices. Technical debt can accumulate over time and make it harder to add new features or fix existing issues. Refactoring can also help code remain relevant as technology evolves, improve code readability, and reduce the complexity of code blocks.
Preparing for Refactoring
As a software engineer, you know that coding is not just about making something work, but also about making it maintainable, easy to read, and scalable. Refactoring is a great way to achieve these goals. Before diving into refactoring your existing codebase, it's important to prepare a plan to ensure the process goes smoothly.
To create a plan for refactoring, first, identify the areas of the codebase that need refactoring and determine the scope of the work. Plan how much time will you spend on each section, and how you will do it. Will you be using a specific refactoring tool, or will you be doing the changes manually? These are essential questions that you need to consider and will help with scheduling tech debt if needed.
Next, establish criteria for successful refactoring. This may include ensuring your code is consistent with naming or commenting conventions, or defining certain performance metrics to measure the success of your refactoring. These criteria will help you maintain focus and ensure the highest quality of code. Be sure to include in your plan a thorough review of the codebase before and after refactoring to identify areas or opportunities for optimization and learn from the experiences you gained through the refactoring process.
While some types of refactoring techniques are low risk and low effort - I highly recommend you separate these out into separate commits in your source control. You'll thank me later.
1. Extract Method Refactoring Technique
By breaking down a long, complex method into smaller units, Extract Method helps eliminate code smell. When the method is smaller, it generally makes code easier to read and maintain. Let's say you have a method that is too long and it's difficult to read. You can extract a smaller part of the method into separate methods and call them from the original method.
The Extract Method technique is a foundational refactoring step that often gets used with more complex refactoring. This is because often we find ourselves needing to move logic out of a particular spot when refactoring. Understanding how to do this effectively will be key to refactoring more complicated scenarios!
2. Replace Conditional with Polymorphism Refactoring Technique
Conditionals can cause code smell, and Replace Conditional with Polymorphism technique replaces repetitive conditional logic with polymorphic objects. Polymorphic objects offer more scalability and flexibility than conditionals, making code more maintainable and efficient. For example, you can use polymorphic objects to replace multiple if/else statements with a single method call to a polymorphic object.
Are you interested in boosting your refactoring skills? Check out my course on Dometrain:
Refactoring for C# Devs: From Zero to Hero
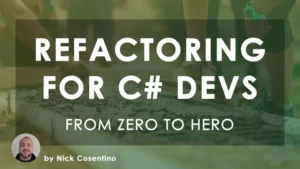
3. Inline Method Refactoring Technique
Inline Method is a technique that is used to get rid of an unnecessary method call. This technique is used to simplify the code by reducing the method calls while still maintaining its functionality. While we often go the other way with the Extract Method technique, there are certain situations where going back can make code more readable.
4. Extract Interface Refactoring Technique
Extract Interface is a technique that is used to define a contract for the class. The interface can then be implemented by multiple classes, making them more versatile and maintainable. This technique is useful when you need to implement a specific behavior in multiple classes or when you need to isolate dependencies.
For example, you can use Extract Interface to create an interface for your data storage class that can be implemented in different classes. If we started with this implementation that worked with SQL:
using System.Data.SqlClient;
public class SqlDataStorage
{
private string _connectionString;
public SqlDataStorage(string connectionString)
{
_connectionString = connectionString;
}
public void SaveData(string data)
{
using (var connection = new SqlConnection(_connectionString))
{
var command = new SqlCommand("INSERT INTO DataTable (Data) VALUES (@Data)", connection);
command.Parameters.AddWithValue("@Data", data);
connection.Open();
command.ExecuteNonQuery();
}
}
public string LoadData()
{
using (var connection = new SqlConnection(_connectionString))
{
var command = new SqlCommand("SELECT TOP 1 Data FROM DataTable ORDER BY Id DESC", connection);
connection.Open();
using (var reader = command.ExecuteReader())
{
if (reader.Read())
{
return reader["Data"].ToString();
}
}
}
return null;
}
}
We could extract the interface as such:
public interface IDataStorage
{
void SaveData(string data);
string LoadData();
}
And then our calling code would rely on this interface instead of the specific implementation. From here, we're able to add alternative implementations, such as support for MongoDB:
using MongoDB.Driver;
using MongoDB.Bson;
public class MongoDataStorage : IDataStorage
{
private IMongoCollection<BsonDocument> _collection;
public MongoDataStorage(string connectionString, string databaseName, string collectionName)
{
var client = new MongoClient(connectionString);
var database = client.GetDatabase(databaseName);
_collection = database.GetCollection<BsonDocument>(collectionName);
}
public void SaveData(string data)
{
var document = new BsonDocument { { "data", data } };
_collection.InsertOne(document);
}
public string LoadData()
{
var sort = Builders<BsonDocument>.Sort.Descending("_id");
var document = _collection.Find(new BsonDocument()).Sort(sort).FirstOrDefault();
return document?["data"].AsString;
}
}
5. Extract Class Refactoring Technique
Extract Class is a technique that helps you separate functionalities by dividing code into different classes. This technique improves the maintainability of your codebase by breaking down a large class into smaller, more manageable classes. For example, you can use Extract Class to divide a large class that handles both database connection and user validation into two classes.
In software development and programming, there's a principle called the Single Responsibility Principle (SRP). And yes, as the name suggests, it's in alignment with what this refactoring technique works towards. Instead of piling logic into one spot, we can organize our code into classes with single responsibilities.
In theory, SRP sounds great to me. However, in practice, I find it nearly impossible to adhere to SRP in any real code base. It's still something handy to strive towards though!
6. Replace Magic Number with Symbolic Constant Refactoring Technique
Magic numbers are hard-coded values that make code difficult to maintain. This technique replaces hard-coded values with symbolic constants, making code easier to read, maintain, and modify. For example, you can use Replace Magic Number with Symbolic Constant to replace a numerical value that represents the maximum number of login attempts with a constant value named MAX_LOGIN_ATTEMPTS.
If you think about it -- at the time you're writing up some code you might very well understand why you're putting 13.9834 into a calculation. Or you might know why you need to go back exactly 7 character positions in your algorithm working with strings. But neither of these numbers are obvious. If we lean into "code being the best documentation", then we can leverage constants with solid naming instead of leaving comments to explain.
7. Replace Inline Code with Function Call Refactoring Technique
This technique is used to improve the readability of the code by replacing repeated inline code with function calls. This makes the code more modular and easier to maintain. It's a variation of the first technique in our list, Extract Method, which aims to accomplish code reusability.
Let's check out an example! If we had the following code, note that we have the substring logic repeated more than once:
class StringProcessor
{
public void Process()
{
string someString = "Hello, this is a sample string";
// Extracting a substring
string extracted = someString.Substring(7, 4);
Console.WriteLine(extracted);
// Some other code...
// Repeating the inline code
extracted = someString.Substring(7, 4);
Console.WriteLine(extracted);
// ...and so on
}
}
We could refactor this to extract the code into a method:
class StringProcessor
{
public void Process()
{
string someString = "Hello, this is a sample string";
// Using a function call
string extracted = ExtractSubstring(someString);
Console.WriteLine(extracted);
// Some other code...
// Repeating the function call
extracted = ExtractSubstring(someString);
Console.WriteLine(extracted);
// ...and so on
}
private string ExtractSubstring(string input)
{
// Centralized logic for substring extraction
return input.Substring(7, 4);
}
}
And bonus question! Which refactoring technique could we apply to clean up our magic numbers? Hint: You've seen it in the list already.
Are you interested in boosting your refactoring skills? Check out my course on Dometrain:
Refactoring for C# Devs: From Zero to Hero
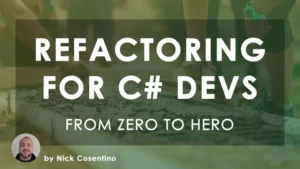
8. Remove Dead Code
Dead code refers to code that is not used or executed and is problematic for software development. We remove unnecessary code to make our codebases more efficient to work in because there's less cognitive load for navigating what's otherwise unused.
Dead code can come up for many reasons. Sometimes when we refactor, code that's no longer called gets left behind. In other situations, developers leave code because they are attached to it and may want to leverage it later.
The first case can be addressed with static analysis tools. These can help find and identify dead code to be cleaned up. The latter case is a cultural shift. Leverage source control tools like git to get code back so that you don't rely on it sticking around unused.
9. Rename Method/Variable
Renaming methods and variables is an important refactoring technique that makes code more readable and easier to maintain. When we think about refactoring, renaming things can greatly improve readability while being very low risk with respect to the type of change. Readability is paramount in programming as we spend the majority of our team reading code and not necessarily just writing it.
It's also important to note that I said very low risk... But it's not zero risk :) Something like reflection could cause you some headaches even with a simple rename!
10. Introduce Design Patterns
Design patterns are proven solutions for commonly occurring problems in software development. This technique helps introduce design patterns in the codebase to solve common problems and improve the maintainability of your codebase.
For example, you can use a Factory Design Pattern to encapsulate object instantiation logic and to make the code more modular. You could look at using a plugin design pattern to introduce more extensibility in an area of your code. Or maybe a facade pattern makes sense to hide complexity after you've refactored code out of a single spot.
Wrapping Up These Top 10 Refactoring Techniques
Refactoring is the process of improving code without changing its functionality (or by changing it minimally but with intention), and it is a crucial part of software development. By refactoring, software engineers and developers can improve the quality, readability, maintainability, and performance of their codebase.
In this article, I have covered a list of the top 10 refactoring techniques that can help transform your codebase. These techniques, including Extract Method, Inline Method, and Introduce Design Patterns, can help to remove code smells, simplify code, and increase its maintainability.
While these techniques are useful, they should not be used blindly just to refactor for the sake of it. Instead, software engineers and developers should carefully consider which of these techniques is appropriate for their codebase. Also consider there's a time and a place to refactor!
I encourage you to apply the techniques learned in this article to improve your codebase continually. Remember to check out my course on Dometrain: Refactoring for C# Devs if you want to skill up on your refactoring! If you'd like to further your learning subscribe to my free weekly newsletter and check out my YouTube channel!
Affiliations
These are products & services that I trust, use, and love. I get a kickback if you decide to use my links. There’s no pressure, but I only promote things that I like to use!
- BrandGhost: My social media content and scheduling tool that I use for ALL of my content!
- RackNerd: Cheap VPS hosting options that I love for low-resource usage!
- Contabo: Affordable VPS hosting options!
- ConvertKit: The platform I use for my newsletter!
- SparkLoop: Helps add value to my newsletter!
- Opus Clip: Tool for creating short-form videos!
- Newegg: For all sorts of computer components!
- Bulk Supplements: Huge selection of health supplements!
- Quora: I answer questions when folks request them!