Working with strings is probably one of the earliest things we get to do as C# developers. In fact, if you consider that most of us start with the "Hello, World!" example, you're being exposed to the string type right away. But as you continue to use strings, you'll quickly find that you want to work with strings that span multiple lines and how we define multiline strings might be a tricky topic for beginners.
No sweat! In this article, we'll look at some simple code examples that demonstrate how to define multiline strings. I'll also link over to GitHub where you can see this code committed and pushed up to a public repository. Finally, the last example contains a special bonus that I think you'll like (even if it's a slightly more advanced topic). Read until the end!
The Base Case (Not a Multi Line String)
This is the most simple example, where we just assign some text between double quotes to a string variable. This is our base case to compare against when we start looking to multi line strings. This example is all declared on a single line, and the resulting string has no line breaks in it either. As easy as it gets!
var simpleString = "Hello, World!";
Console.WriteLine(simpleString);
As you might expect, the output looks something just like this:
Hello, World!
Multiline Strings in One Line of Code
Our next example is perhaps the most simple way to multi line a string. However, it is important to note that this example looks at creating a multiline string where the resulting value of the string is across multiple lines. The string itself in code is still all defined on one line
var multilineString = "Hello,rnWorld!";
Console.WriteLine(multilineString);
This example shows the carriage return (r) and linefeed (n) characters added into the string to make the output span across two lines. These line endings can be different on Windows vs unix so consider using Environment.NewLine. It's also important to note that we use the backslash here which makes it an escape character (and that's why you don't see an extra r and n in the console output):
Hello,
World!
Multiline Strings in Multiple Lines of Code
This is much like the previous example, except we will use the + operator to concatenate the strings. This string is now across multiple lines in our code AND multiple lines in the output.
var multilineString =
"Hello,rn" +
"World!";
Console.WriteLine(multilineString);
Because we have two constant strings being concatenated together, the compiler will treat these as one single string for us. However, it afforded us the ability to split the string across multiple lines when we created it so that it was perhaps easier to read.
The output is exactly the same as the previous example:
Hello,
World!
Single Line String Across Multiple Lines of Code
Now we're on a roll. So what happens if we want to be able to use the string concatenation operator (+) but we don't want the result to be a multiline string? Simple! We can remove our new line characters r and n.
var stringAcrossMultipleLines =
"Hello" +
"," +
" " +
"World" +
"!";
Console.WriteLine(stringAcrossMultipleLines);
The output of this will therefore print to one single line in the console as follows:
Hello, World!
Multiline Strings as Verbatim Strings
This example gets a little bit fancier! I would argue that this is probably (until recently) one of the more popular ways to declare multiline strings in your code. It gives you the benefit of having multi line strings with respect to their resulting value, but they also easily span multiple lines of code without having to put a string concatenation operator (+) at the end of every line.
Let's look at the example:
var verbatimString = @"Hello,
world! I wonder if this
string will have funny
gaps even though it
looks like it is
aligned to the same
indentation in the editor...";
Console.WriteLine(verbatimString);
Console.WriteLine();
The @ symbol at the start of the string definition (i.e. right before the double quotes) marks the string as verbatim. This example is a little bit contrived, because I wanted to illustrate one of the pitfalls of this method if you're not aware of it. Verbatim strings will include all of your indentation as part of the actual string itself! Sometimes this might be what you expect (truly, it is verbatim) and other times it might be a bit frustrating.
Here's the output:
Hello,
world! I wonder if this
string will have funny
gaps even though it
looks like it is
aligned to the same
indentation in the editor...
A New Challenger Approaches: Raw String Literals
In C# 11 we get access to this new fancy feature called raw string literals. This might be old news if you have seen other programming languages with similar functionality, but it's big news for us C# developers. This is super neat for supporting multi line strings in C#.
I view the raw string literal option as being much like a verbatim string, but without the worry about indentation. We can denote a raw string literal with triple quotes, and if we have a string with triple quotes inside of it already, we can use four quotes as well. This pattern repeats based on how many quotes you need to support.
Let's check out the example:
var rawStringLiteral =
// Note: try playing with the indentation of all of the lines here
"""
"Hello,
world!" I wonder if this
string will have funny
gaps even though it
looks like it is
aligned to the same
indentation in the editor...
Maybe just
these lines
right here.
""";
Console.WriteLine(rawStringLiteral);
In the example above, we have DOUBLE indented the multi line string, but the Visual Studio IDE will actually give you a visual indicator as to where the start of the line actually is. In this case, it's directly in line with the left side of the triple quotes.
That means our output is going to look like this:
"Hello,
world!" I wonder if this
string will have funny
gaps even though it
looks like it is
aligned to the same
indentation in the editor...
Maybe just
these lines
right here.
It's important to note only the last few lines were actually indented, even though all of the lines were indented in the code. That's just because of where the alignment gets set with the raw string literals. In my opinion, something I will be using a TON of for my multiline strings.
Here's the output in the console from all of our examples:
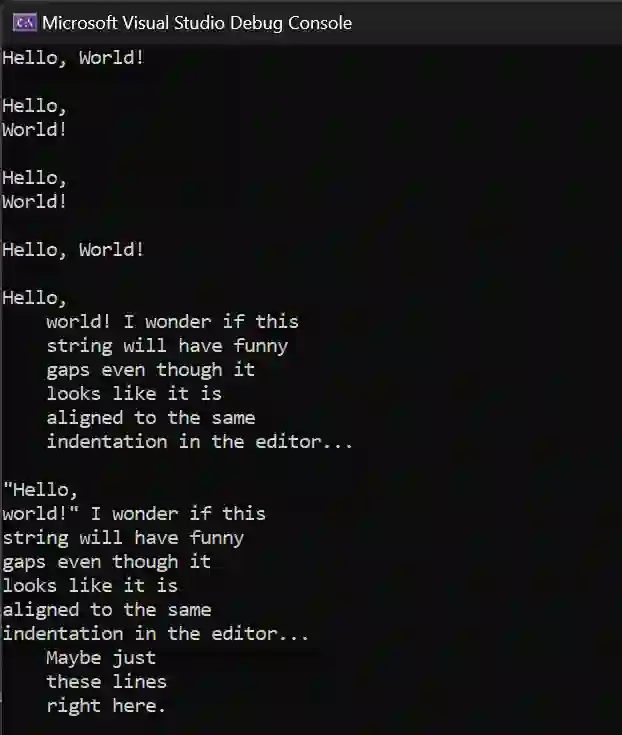
Bonus Round - Special Access for Raw String Literals
Alright, I did promise a bonus at the start of this article and I wasn't lying. Raw string literals are a feature that comes in C# 11. But what happens if you are targeting an older version of .NET and want to access this feature?
Fortunately, I have covered this topic in a previous article, but we can elaborate on it here too. Essentially, we can use a nuget package called PolySharp and set the LangVersion attribute in the csproj file to be 11.0. If you have your build machine setup with C# 11 requirements, it will allow you to compile the code for raw string literals on older framework versions.
Don't believe me? You can prove it for yourself when you go look at the code I have pushed up to GitHub for this article!
Wrapping Up Multi Line Strings in CSharp
Strings are an essential building block for all of us C# developers and other programming languages for that matter. Creating a multiline string is a common occurrence for many of us, especially if you're formatting things for humans to read.
Depending on your skill level and familiarity, you might not know the various ways you can create a multiline string in C#. It's also important to decide whether or not you want to have a multiline string with respect to how it looks in code, how the result value is actually formatted, or both! The methods in this article should help you decide and start writing tons of multiline strings!
Frequently Asked Questions: Wrapping Up Multi Line Strings in C#
\u003cstrong\u003eWhat are multiline strings in C# and how do you create them?\u003c/strong\u003e
Multiline strings in C# allow text to span multiple lines within the code. They can be created using verbatim strings with the @
symbol or with the new C# 11 feature called raw string literals using triple quotes """
.
\u003cstrong\u003eHow can you include line breaks in a C# string without using multiple lines of code?\u003c/strong\u003e
Line breaks can be included in a single line of C# code by using escape sequences, such as \r\n
(carriage return + line feed) within the string.
\u003cstrong\u003eWhat are verbatim strings in C# and when should you use them?\u003c/strong\u003e
Verbatim strings, marked by the @
symbol before the string, allow for multiline strings and ignore escape sequences, making them ideal for file paths and regular expressions.
\u003cstrong\u003eCan you concatenate multiline strings in C#?\u003c/strong\u003e
Yes, multiline strings can be concatenated using the +
operator, allowing for complex strings to be built across multiple lines of code.
\u003cstrong\u003eWhat are raw string literals in C# 11, and how do they improve string handling?\u003c/strong\u003e
Raw string literals in C# 11, denoted by triple quotes """
, simplify multiline string creation without worrying about indentation affecting the string content, enhancing readability and maintainability.
\u003cstrong\u003eHow do you handle indentation in multiline strings with C# verbatim strings?\u003c/strong\u003e
Indentation in verbatim strings is included in the string content, which can lead to unexpected spacing. Care must be taken to ensure the desired formatting.
\u003cstrong\u003eWhat is the significance of using \u003ccode\u003eEnvironment.NewLine\u003c/code\u003e in C# strings?\u003c/strong\u003e
Environment.NewLine
is used to ensure that the newline character(s) used are appropriate for the operating system the application is running on, providing platform-independent line breaks.
\u003cstrong\u003eHow do raw string literals handle quotes and indentation in C# 11?\u003c/strong\u003e
Raw string literals allow for flexible handling of quotes and indentation, where the starting indentation level is determined by the position of the triple quotes and additional quotes can be managed by adding more quotes as needed.
\u003cstrong\u003eWhat are the best practices for creating and using multiline strings in C# applications?\u003c/strong\u003e
Best practices include choosing the right method (escape sequences, verbatim strings, or raw string literals) based on the context, being mindful of indentation and platform-specific newline characters, and using concatenation wisely to maintain readability.